Node.js has emerged as a popular choice for building web applications due to its event-driven, non-blocking I/O model. Asynchronous programming lies at the heart of Node.js, empowering developers to create highly scalable and performant applications. In this technical post, we will explore how to supercharge your Node.js development by harnessing the power of asynchronous programming, scalability, and real-time communication, enabling you to unlock the full potential of Node.js for high-performance web applications and microservices.
Understanding Asynchronous Programming in Node.js
Asynchronous programming lies at the core of Node.js, and it is a fundamental concept that sets it apart from traditional server-side frameworks. This approach to handling tasks allows Node.js to efficiently manage multiple operations simultaneously without blocking the main event loop.
In this in-depth exploration of asynchronous programming in Node.js, we’ll delve into the mechanics, benefits, and best practices, enabling developers to harness its power and build high-performance, scalable applications.
1. The Event Loop and Non-Blocking I/O
At the heart of Node.js’s asynchronous nature is the event loop. The event loop is a central component that enables the execution of asynchronous tasks while efficiently managing other concurrent tasks. It continuously runs in the background, processing events and callbacks, ensuring that tasks can be completed without waiting for each other.
When an asynchronous task, such as reading from a file or making an API call, is initiated in Node.js, it does not block the entire application from continuing with other tasks. Instead, Node.js delegates the task to the underlying operating system, which handles it asynchronously. Once the task is completed, a callback function is placed in the callback queue.
The event loop’s job is to continuously check the callback queue and execute the callbacks of completed tasks, moving them to the call stack for processing. This process ensures that Node.js can handle multiple operations concurrently, providing a highly efficient and scalable environment for web applications and real-time communication.
2. Callbacks, Promises, and async/await
In asynchronous programming, there are various ways to handle the flow of execution. Traditionally, callbacks were the primary method used in Node.js. A callback function is passed as an argument to an asynchronous function, and it is called once the operation is completed. While callbacks are functional, they can lead to complex and nested structures, known as “callback hell,” making code difficult to read and maintain.
To alleviate the issues of callback hell, Promises were introduced in Node.js. Promises provide a more structured way of handling asynchronous operations. A Promise is an object that represents the eventual completion (or failure) of an asynchronous operation and allows developers to chain multiple asynchronous tasks together elegantly.
Building on Promises, the async/await syntax was introduced in recent versions of Node.js. async/await simplifies asynchronous programming even further by allowing developers to write asynchronous code that looks and behaves like synchronous code. It eliminates the need for explicit chaining of Promises and makes the flow of execution more intuitive.
3. Handling Errors in Asynchronous Code
Error handling is a critical aspect of asynchronous programming, as asynchronous tasks can result in unforeseen errors. In traditional callback-based approaches, error handling could become convoluted and challenging to manage. However, with Promises and async/await, error handling has become more structured and straightforward.
Promises provide a built-in .catch()
method to handle errors that occur during asynchronous operations. Similarly, in async/await syntax, try/catch blocks are used to catch errors from asynchronous operations within the same scope. This makes error handling more robust and enhances code reliability.
Implementing Asynchronous APIs
Creating well-designed asynchronous APIs is crucial for Node.js development. We will cover best practices for designing APIs that respond quickly to requests and efficiently handle concurrent user interactions. Proper error handling, callback patterns, and graceful handling of asynchronous code will be discussed to ensure robust and reliable API endpoints.
Scalability with Node.js Clustering and Load Balancing
As your application grows, scalability becomes a key concern. Node.js provides tools such as clustering and load balancing to distribute the application workload across multiple cores and machines. By harnessing these techniques, you can achieve horizontal scalability, allowing your application to handle an increasing number of users and optimize resource utilization.
Real-Time Communication with WebSockets
Node.js excels in real-time communication scenarios. We will dive into WebSockets, a powerful protocol that enables bi-directional, low-latency communication between clients and servers. Discover how to integrate WebSockets into your Node.js application to build interactive and responsive real-time features, such as chat applications, live notifications, and collaborative tools.
Caching and In-Memory Databases for Faster Data Access
Efficient data retrieval is essential for web applications. We will explore how to implement caching mechanisms and leverage in-memory databases like Redis to reduce latency and speed up data access. By employing caching strategies, you can enhance user experience and significantly reduce server load, resulting in a more responsive application.
Security Best Practices for Node.js
Node.js is a powerful and versatile platform, but like any other technology, it requires diligent attention to security to protect applications and data from potential threats. As Node.js gains popularity, it becomes an attractive target for attackers. In this comprehensive guide, we will explore essential security best practices for Node.js development, enabling developers to build robust and resilient applications.
1. Keep Node.js and Dependencies Up-to-Date
Regularly update Node.js to the latest stable version to benefit from the latest security patches and bug fixes. As the Node.js community actively identifies and addresses security vulnerabilities, staying up-to-date is crucial in safeguarding your application against known threats.
Dependency management is equally important. Dependencies are the building blocks of your Node.js project, and outdated or vulnerable packages can be exploited by attackers. Use package managers like npm or yarn to manage your project’s dependencies efficiently. Leverage tools like npm audit to identify and address vulnerable packages, ensuring that you use secure versions of libraries.
Moreover, consider enabling automatic updates for your dependencies to receive security fixes promptly. Keeping your Node.js and dependencies up-to-date is a proactive measure that significantly reduces your application’s attack surface and enhances overall security.
2. Implement Input Validation
Web applications are exposed to a wide range of security risks, particularly from user input. Malicious actors can exploit input fields to execute code, perform SQL injections, or launch cross-site scripting (XSS) attacks. Implementing robust input validation is a crucial defense against such attacks.
Always validate and sanitize user input on both the client and server sides. Use regular expressions and input validation libraries to ensure that incoming data matches the expected format and meets specific criteria. Reject or sanitize any input that doesn’t comply with the predefined validation rules.
Furthermore, avoid concatenating user input directly into SQL queries or HTML templates. Instead, use parameterized queries for database operations and escape output to prevent XSS attacks. By applying proper input validation and sanitization, you can prevent a wide range of security vulnerabilities and enhance the overall security posture of your Node.js application.
3. Enforce Authentication and Authorization
Authentication and authorization are cornerstones of web application security. Properly implementing user authentication ensures that only legitimate users can access your application’s resources.
Use strong and modern authentication mechanisms, such as OAuth 2.0 or JSON Web Tokens (JWT), to verify user identities. Avoid storing plaintext passwords; instead, use strong password hashing algorithms like bcrypt to store user passwords securely.
Once authenticated, enforce proper authorization checks to control what authenticated users can access within your application. Implement role-based access control (RBAC) to define specific permissions for different user roles. By enforcing both authentication and authorization, you limit potential attack vectors and protect sensitive data from unauthorized access.
Performance Monitoring and Optimization
To ensure your Node.js application maintains peak performance, monitoring and optimization are essential. We will explore various tools and techniques to profile and analyze your application’s performance, identify bottlenecks, and optimize code and configurations for optimal execution.
Deploying Node.js Applications for Production
A well-developed Node.js application needs a robust deployment strategy. We will discuss considerations for deploying Node.js applications in a production environment, including load balancing, containerization with Docker, monitoring with tools like Prometheus, logging, and automated deployment processes with CI/CD pipelines.
Conclusion
Node.js offers a powerful platform for building high-performance web applications and microservices. By mastering asynchronous programming, scalability techniques, and embracing real-time communication, you can supercharge your Node.js development efforts and deliver efficient, responsive, and scalable applications.
Embrace the event-driven, non-blocking paradigm of Node.js, and explore the vast ecosystem of tools and libraries to take your Node.js development to new heights. With the right approach and commitment to providing value, you can elevate your applications, foster lasting relationships with users, and thrive in the dynamic and competitive world of web development.
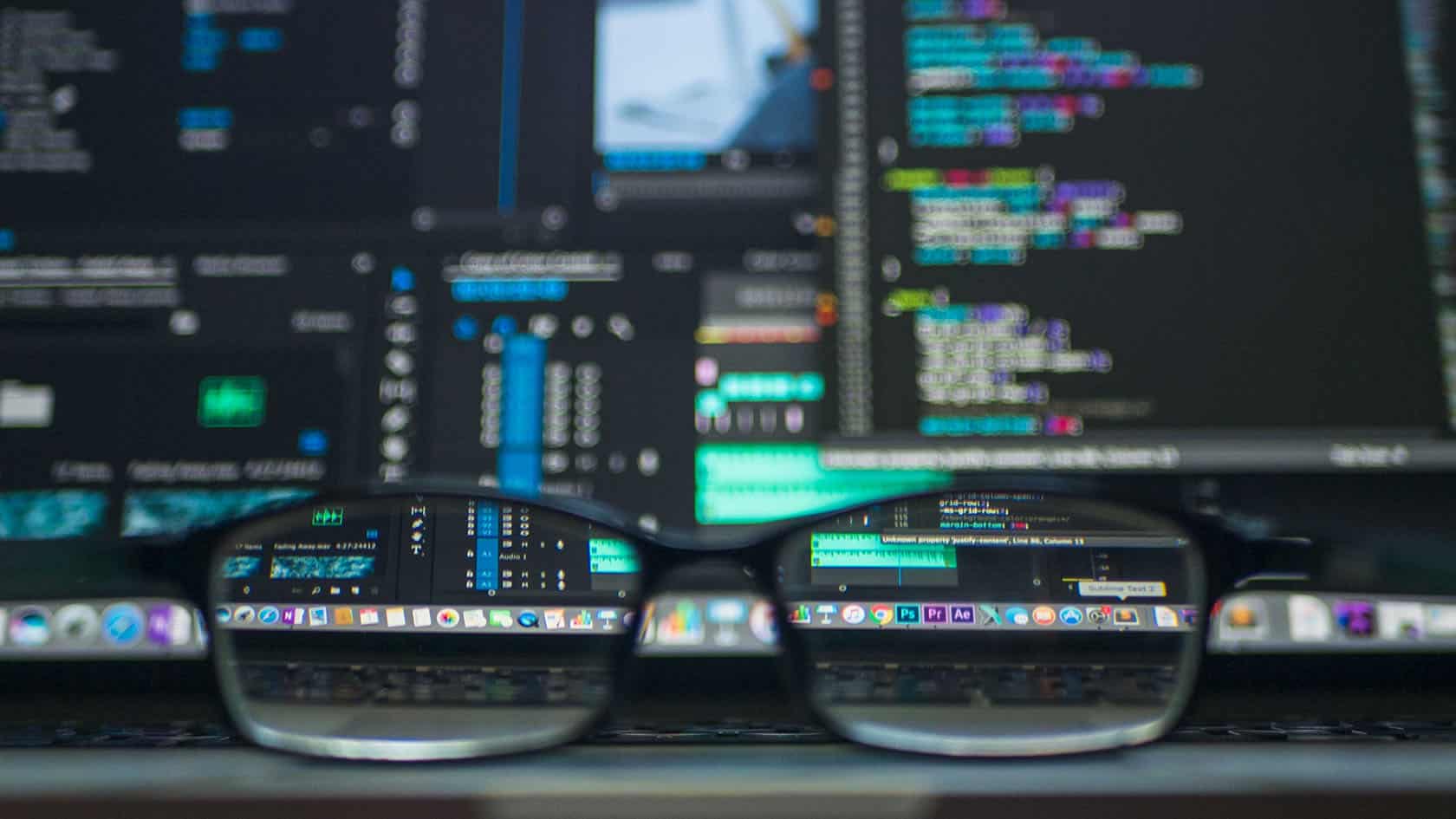